Background
The process of obtaining a customers usage data takes time, the duration to obtain the latest extract can take up to 2 weeks (despite the Electricity Authority requiring them to provide in no more than 5 days). The snapshots API is an automated mechanism to request the snapshot for a customer and then subsequently getting the snapshot to generate insights. The steps are as follows:
- Customer provides (from their electricity provider) a .csv file (or a collection of files) of their energy usage (via snapshots POST API call)
- Ahiko converts the received .csv file into a standard, clean usage format, making it available for insight generation (via snapshots GET API call)
Prerequisites
Before you can follow this guide, you will need:
- To be able to make a request in your programming language of choice, our API reference has example requests using multiple common languages and libraries, just look for the "Language" dropdown at the top of the page.
- Your subscription key (32 alphanumerical combination key).
Snapshots (POST & GET)
Both API calls are may via the url https://api.ahiko.io/usage/v1/snapshots but depending on the need it can be called in the POST or GET form. Let's break them down:
POST - Initiating a Snapshot Request
Using your language of choice, make a POST request to the https://api.ahiko.io/usage/v1/snapshots endpoint, with the following parameters:
--header ahiko-app-id: {{application subscription key}}
Where the {{application subscription key}}
is replaced with your application subscription key.
In addition, given we are uploading 1 or many files from a vendor in a raw format, and as such the we use a multipart form-data to capture the uploaded file(s), and as such the following parameters are also required
--header 'content-type: multipart/form-data'
--form customer_id=customer_def \
--form property_id=property_ghi \
--form raw_electricity_provider_file=@powernewco1_demo.csv
--form raw_electricity_provider_file=@powernewco2_demo.xlsx
The reason we allow for multiple provider files in one snapshot is to accommodate scenarios where a household has moved between 2 or more vendors during the period of insights. However, it's common to only provide one. Either way the consolidated, normalised data that is generated, is one file, consider it a merge of all the data in the files for the same ICP address.
POST - Handling the Response
The Ahiko API uses JSON to encode responses. Most languages have libraries to encode and decode JSON.
The API response will look like:
{
"success": true,
"id": "task_d5c5b3a8-2174-48b1-ba33-56dfca574bbf"
}
Let's break this response down:
success
is true. That means that the request was successfulid
is a str. This is the unique identifier for the task requested, it can be used to subsequently get the snapshot as the unique identifier. It's important the calling client holds onto this.
GET - Retrieving the Snapshot
Using your language of choice, make a GET request to the https://api.ahiko.io/usage/v1/snapshots/{task_id} endpoint, with the following parameters:
--header ahiko-app-id: {{application subscription key}}
Where the {{application subscription key}}
is replaced with your application subscription key and where the {{task_id}}
is the name of the task resource.
GET - Handling the Response
The API response will look different depending on the snapshot_lifecycle
. Lets explain further. A snapshot has a lifecycle that can be any one of 3 states processing | succeeded | failed
. The following sequence diagram explains the flow between these states:
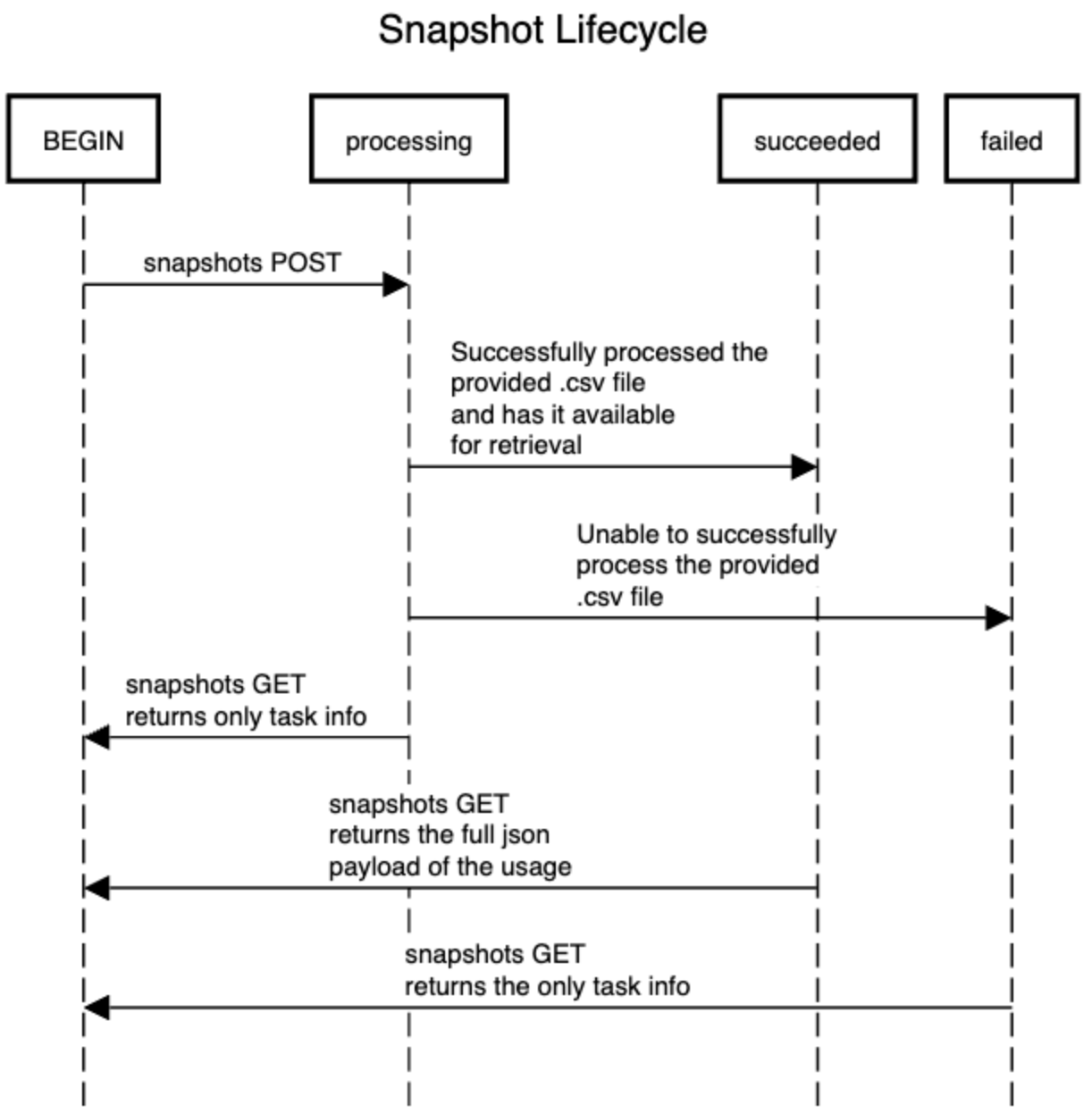
GET Response for "processing" snapshot_lifecycle
snapshot_lifecycle
{
"success":true,
"data":{
"id": "d5c5b3a8-2174-48b1-ba33-56dfca574bbf",
"snapshot_lifecycle": "processing",
"created_at": "2023-09-27T00:52:37.906836Z",
"updated_at": "2023-09-27T00:52:37.906836Z",
"task":{
"customer_id": "customer_abceefefefefefe123",
"property_id": "property_def456",
"electricity_provider_filename": [
"trustpower_sample0001_onemonth.csv",
"mercury_sample0002_onemonth.csv"
],
}
}
}
GET Response for "succeeded" snapshot_lifecycle
snapshot_lifecycle
{
"success":true,
"data":{
"id": "d5c5b3a8-2174-48b1-ba33-56dfca574bbf",
"snapshot_lifecycle": "succeeded",
"created_at": "2023-05-01T21:02:08.176249Z",
"updated_at": "2023-05-04T09:02:08.176249Z",
"task":{
"customer_id": "customer_abceefefefefefe123",
"property_id": "property_def456",
"electricity_provider_filename": [
"trustpower_sample0001_onemonth.csv",
"mercury_sample0002_onemonth.csv"
],
"electricity_provider_filename": "trustpower_sample0001_onemonth.csv",
"normalised_electricity_provider_file_blob_url": "normalised_electricity_provider_file_blob_url"
}
}
}
GET Response for "failed"snapshot_lifecycle
snapshot_lifecycle
{
"success":true,
"data":{
"id": "d5c5b3a8-2174-48b1-ba33-56dfca574bbf",
"snapshot_lifecycle": "failed",
"failure_reason":"Unsupported format",
"created_at": "2023-05-01T21:02:08.176249Z",
"updated_at": "2023-05-04T09:02:08.176249Z",
"task":{
"customer_id": "customer_abceefefefefefe123",
"property_id": "property_def456",
"electricity_provider_filename": [
"trustpower_sample0001_onemonth.csv",
"mercury_sample0002_onemonth.csv"
],
}
}
}
Reading Blobs - Normalised Usage
The snapshots generate blobs to hold the normalised usage data. This is a publicly accessible file url that the client and use to efficiently download the usage payload. The format of this file is a .json file.
{
..
"normalised_electricity_provider_file_blob_url": "normalised_electricity_provider_file_blob_url"
}
If you inspect the contents of the normalised_electricity_provider_file_blob_url
you will see the following format:
Blob File Format
[
{
"date": "2022-09-01",
"time": "00:00",
"kwh": 0.11
},
{
"date": "2022-09-01",
"time": "00:30",
"kwh": 0.12
},
...
]
Let's break this blob contents:
date
is a yyyy-MM-dd format of the usage datetime
is HH:mm format of the time of the observed usagekwh
is the actual usage over that period in kWh
Handling Errors
The Ahiko API returns standard HTTP response codes.
Below are the most common error codes and their reasons:
200
Everything is OK. The response is included in the body.404
The url does not exist. Check you have entered the url correctly.403
You are not allowed to access this resource. Make sure you have a valid user access token with the correct permissions. Also make sure you have set the Authorization header correctly (note the American spelling).404
The specified resource does not exist
You should treat any response code that is greater than 399 as an error.